11.30.08
Posted in Hacking, Maps, Too Much Information at 11:21 pm by ducky
Warning: this is a long and geeky post.
From time to time in the past few years, I have mentioned that I was a little puzzled as to why more people didn’t render tiles on-the-fly for Google Maps, as I do in my U.S. Census Bureau/Google Maps mashup.
I have reappraised my attitude. I have been redoing my mapping framework to make it easier to use. I have reminded myself of all the hurdles I had to overcome, and discovered a number of annoying new ones.
First pass
I originally wrote my mapping framework in an extreme hurry. It was a term project, and a month before the end of the term, I realized that it would be good for personal reasons to hand it in a week early. The code functioned well enough to get me an A+, but I cut a huge number of corners.
Language/libraries/database choice
It was very important to minimize risk, so I wrote the framework in C++. I would have liked to use a scripting language, but I knew that I would need to use a graphics library and a library to interpret shapefiles. The only ones I found that looked reasonable were C-based libraries (Frank Warmerdam’s Shapelib library andThomas Boutell’s gd library). I knew it was possible using a tool called SWIG, but I hadn’t ever used SWIG and had heard that it was touchy. Doing it in C++ was guaranteed to be painful, but I knew what the limits of that pain were. I didn’t know what the limits of pain of using SWIG would be.
Projection
I also had problems figuring out how to convert from latitude/longitude to pixel coordinates in the Google tile space. At the time (December 2005), I had a hard time simply finding out what the mathematics of the Mercator transformation were. (It is easier to find Mercator projection information now.) I was able to figure out something that worked most of the time, but if you zoomed out past a certain level, there would be a consistent error in the y-coordinates. The more you zoomed out, the bigger the error. I’m pretty sure it’s some sort of rounding error. I looked at it several times, trying to figure out where I could possibly have a roundoff error, but never did figure it out. I just restricted how far people could zoom out. (It also took a very long time to render tiles if you were way zoomed out, so it seemed reasonable to restrict it.)
Polygon intersection
I remember that I spent quite a lot of time on my polygon intersection code. I believe that I looked around the Web and didn’t find any helpful code, so developed it from scratch on little sleep. (Remember, I was doing this in a frantic, frantic hurry.) I ended up with eight comparisons that needed to be done for each polygon in the database for every tile. More on this later.
Rendering bug
The version I handed in had a bug where horizontal lines would show up at the bottom of tiles frequently, as you can see in the bottom left tile:

It was pretty obvious that the bug was my fault, as gd is a very mature and well-used graphics library. My old office partner Carlos Pero had used it way back in 1994 to develop Carlos’ Coloring Book, so it was clear to me that the problem was my fault.
After I handed in my project, I spent quite a lot of time going through my code trying to figure out where the problem was with no luck. Frustrated, I downloaded and built gd so that I could put breakpoints into the gd code. Much to my surprise, I discovered that the bug was in the gd library! I thus had to study and understand the gd code, fix it, report the bug (and patch), and of course blog about it so that other people wouldn’t have the same problem.
Pointing my code to the fixed gd
Then, in order to actually get the fix, I had to figure out how to statically link gd into my binaries. I like my ISP (Dreamhost) and wasn’t particularly interested in changing, but that meant I couldn’t use the system-installed gd libraries. Statically linking wasn’t a huge deal, but it took me at least several hours to figure out which flag to insert where in my makefile to get it to build statically. It was just one more thing.
Second pass
I have graduated, but haven’t found a job yet, so I decided to revamp my mapping framework. In addition to the aesthetic joy of making nice clean code:
- It would be an opportunity to learn and demonstrate competence in another technology.
- I had ideas for how I could improve the performance by pre-computing some things.
- With a more flexible framework, I would be able to do some cool new mashups that I figured would get me more exposure, and hence lead to some consulting jobs.
Language/libraries/database choice
Vancouver is a PHP town, so I thought I’d give PHP a shot. I expected that I might have to rewrite my code in C++ eventually, but that I could get the basics of my improved algorithms shaken out first. (I’m not done yet, but so far, I have been very very pleased with that strategy.)
I also decided to use MySQL. While the feeling in the GIS community is that the Postgres‘ GIS extensions (PostGIS) are better than the GIS extensions to MySQL, I can’t run Postgres on my ISP, and MySQL is used more than Postgres.
I had installed PHP4 and MySQL 4 on my home computer some time ago, when I was working on Mapeteria. However, I recently upgraded my home Ubuntu installation to Hardy Heron, and PHP4 was no longer supported. That meant I need to install a variety of packages, and I went through a process of downloading, trying, discovering I was missing a package, downloading/installing, discovering I was missing a package, lather, rinse, repeat. I needed to install mysql-server-5.0, mysql-client-5.0, php5, php5-mcrypt, php5-cli, php5-gd, libgd2-xpm-dev, php5-mysql, and php5-curl. I also spent some time trying to figure out why php5 wouldn’t run scripts that were in my cgi-bin directory before realizing/discovering that with mod_php, it was supposed to run from everywhere but the cgi-bin directory.
Note that I could have done all my development on my ISP’s machines, but that seemed clunky. I knew I’d want to be able to develop offline at some point, so wanted to get it done sooner rather than later. It’s also a little faster to develop on my local system.
I did a little bit of looking around for a graphics library, but stopped when I found that PHP had hooks to the gd library. I knew that if gd had not yet incorporated my horizontal lines bug fix, then I might have to drop back to C++ in order to link in “my” gd, but I figured I could worry about that later.
Projection
I made a conscious decision to write my Mercator conversion code from scratch, without looking at my C++ code. I did this because I didn’t want to be influenced in a way that might lead me to get the same error at way-zoomed-out that I did before. I was able to find equations on the Wikipedia Mercator page for transforming Mercator coordinates to X-Y coordinates, but those equations didn’t give a scale for the X-Y coordinates! It took some trial and error to sort that out.
Data
For the initial development, I decided to use country boundaries instead of census tract boundaries. The code wouldn’t care which data it was using, and it would be nice to have tiles that would render faster when way-zoomed-out. I whipped up a script read a KML file with country boundaries (that I got from Valery Hronusov and used in my Mapeteria project) and loaded it into MySQL. Unfortunately, I had real problems with precision. I don’t remember whether it was PHP or MySQL, but I kept losing some precision in the latitude and longitude when I read and uploaded it. I eventually converted to uploading integers that were 1,000,000 times the latitude and longitude, and so had no rounding difficulties.
One thing that helped me enormously when working on the projection algorithm was to gather actual data via Google. I found a number of places on the Google maps where three territories (e.g. British Columbia, Alberta, and Montana) came together. I would determine the latitude/longitude of those points, then figure out what the tile coordinates, pixel X, and pixel Y of that point were for various zoom levels. That let me assemble high-quality test cases, which were absolutely essential in figuring out what the transformation algorithm should be, but it was very slow, boring, and tedious to collect that data.
Polygon intersection
When it came time to implement my polygon bounding box intersection code again, I looked at my old polygon intersection code again, saw that it took eight comparisons, and thought to myself, “That can’t be right!” Indeed, it took me very little time to come up with a version with only four comparisons, (and was now able to find sources on the Web that describe that algorithm).
Stored procedures
One thing that I saw regularly in employment ads was a request for use of stored procedures, which became available with MySQL 5. It seemed reasonable that using a stored procedure to calculate the bounding box intersection would be even faster, so I ran some timing tests. In one, I used PHP to generate a complex WHERE clause string from eight values; in the other, I passed eight values to a stored procedure and used that in the WHERE clause. Much to my suprise, it took almost 20 times more time to use the stored procedure! I think I understand why, but it was interesting to discover that it was not always faster.
GIS extensions
My beloved husband had been harping on me to use the built-in GIS extensions. I had been ignoring him because a large part of the point of this exercise was to learn more about MySQL, including stored procedures, but now that I found that the stored procedure was slow, it was time to time the built-in bounding box intersection routine. If I stored the bounding box as a POLYGON type instead of as two coordinate pairs, then calculating the intersection took half the time. Woot!
Rendering
I discovered that despite my having reported the horizontal lines bug fifteen months ago, the gd team hasn’t done anything with it yet. Needless to say, this means that the version of libgd.a on Dreamhost has the bug in it. I thought about porting back to C++. I figured that porting back would probably take at minimum a week, and would raise the possibility of nasty pointer bugs, so it was worth spending a few days trying to get PHP to use my version of gd.
It is possible to compile your own version of PHP and use it, though it means using the CGI version of PHP instead of mod_php. I looked around for information on how to do that, and found a Dreamhost page on how to do so.. but failed utterly when I followed the directions. I almost gave up at that point, but sent a detailed message to Dreamhost customer support explaining what I was trying to do, why, and what was blocking me. On US Thanksgiving Day, I got a very thoughtful response back from Robert at Dreamhost customer support which pointed me at a different how-to-compile-PHP-on-Dreamhost page that ultimately proved successful. (This is part of why I like Dreamhost and don’t really want to change ISPs.)
Compiling unfamiliar packages can be a real pain, and this was no different. The Dreamhost page (on their user-generated wiki) had a few scripts that would do the install/build for me, but they weren’t the whole story. Each of the scripts downloaded a number of projects (like openSSL, IMAP, CURL, etc) in compressed form, extracted the files, and built them. The scripts were somewhat fragile — they would just break if something didn’t work right. They were sometimes opaque — they didn’t always print an error message if something broke. If there was a problem, they started over from the beginning, removing everything that had been downloaded and extracted. Something small — like if the mirror site for mcrypt was so busy that the download timed out — would mean starting from scratch. (I ended up iteratively commenting out large swaths of the scripts so that I wouldn’t have to redo work.)
There was some problem with the IMAP build having to do with SSL. I finally changed one of the flags so that IMAP built without SSL — figuring that I was unlikely to be using this instance of PHP to do IMAP, let alone IMAP with SSL — but it took several false starts, each taking quite a long time to go through.
Finally, once I got it to build without my custom gd, I tried folding in my gd. I uploaded my gd/.libs directory, but that wasn’t enough — it wanted the gd.h file. I suppose I could have tried to figure out what it wanted, where it wanted it, but I figured it would be faster to just re-build gd on my Dreamhost account, then do a make install to some local directory. Uploading my source was fast and the build was slow but straightforward. However, I couldn’t figure out how to specify where the install should go. The makefiles were all autogenerated and very difficult to follow. I tried to figure out where in configure the install directory got set, but that too was hard to decipher. Finally, I just hand-edited the default installation directory. So there. That worked. PHP built!
Unfortunately, it wouldn’t run. It turned out that the installation script had a bug in it:
cp ${INSTALLDIR}/bin/php ${HOME}/${DOMAIN}/cgi-bin/php.cgi
instead of
cp ${INSTALLDIR}/bin/php.cgi ${HOME}/${DOMAIN}/cgi-bin/php.cgi
But finally, after all that, success!

Bottom line
So let me review what it took to get to tile rendering on the second pass:
- Choose a database and figure out how to extract data from it, requiring reading and learning.
- Find and load boundary information into the database, requiring trial and error.
- Choose a graphics library and figure out how to draw coloured polygons with it, requiring reading and learning.
- Gather test cases for converting from latitude/longitude into Google coordinate system, requiring patience. A lot of patience.
- Figure out how to translate from latitude/longitude pairs into the Google coordinate system, requiring algorithmic skills.
- Diagnose and fix a bug in a large-ish C graphics library, requiring skill debugging in C.
- Download and install PHP and MySQL, requiring system administration skills.
- Figure out how to build a custom PHP, requiring understanding of bash scripts and makefiles.
So now, I guess it isn’t that easy to generate tiles!
Note: there is an entirely different ecosystem for generating tiles, one that comes from the mainline GIS world, one that descends from the ESRI ecosystem. I expect that I could have used PostGIS and GeoTools with uDig look like fine tools, but they are complex tools with many many features. Had I gone that route, I would have had to wade through a lot of documentation of features I didn’t care about. (I also would have had to figure out which ISP to move to in order to get Postgres.) I think that it would have taken me long enough to learn / install that ecosystem’s tools that it wouldn’t have been worth it for the relatively simple things that I needed to do. Your milage may vary.
Permalink
11.27.08
Posted in Politics at 9:27 am by ducky
One of the things I really like about Obama is what, for lack of a better term, I will call middle-class values. He does things like clean up after himself at an ice cream shop, carry his own luggage (pictures here and here and here) and says he turns off lights and will make his kids do chores in the White House. I don’t recall ever seeing any of the presidents in my adult lifetime — Reagan, Bush 41, Clinton, or Bush 43 — ever carrying anything, even when they were campaigning. I suspect that Bush 41 never washed a dish or picked up dog poop — ever. I can’t imagine that either of the Clintons would do so now.
People in power frequently have other people do mundane things for them. There is a potential that, by doing things himself, Obama could make himself seem less powerful. Jimmy Carter once spent the night in a private home, and it was reported in all the newspapers that he made the bed himself. My recollection of that is that people were kind of incredulous at him diminishing himself that way. However, Jimmy Carter ran with a persona of folksiness. (He was Jimmy Carter, not James Earl Carter, Jr.) He had to struggle a little against being perceived as a rube, a southern bumpkin.
I don’t think Obama really risks debasing himself in the public eye by doing mundane things for himself. In contrast to Jimmy Carter, Obama has a public persona that is a bit cold and standoffish. He even got attacked for being elitist for a little while. Doing mundane things for himself counters that perception.
Maybe he carefully does these mundane things for show. Maybe he’s conscious of it and wants to “keep it real”. But maybe it’s part of his value system that he is not inherently better than other people, and should play by the same rules as the rest of the world. (Unlike, say, Arnold Schwarzenegger, who has demonstrated a pattern throughout his life of acting like rules were for other people.) I hope so.
Permalink
11.26.08
Posted in Politics at 9:47 pm by ducky
There has been discussion about how the current financial system bailout is the most expensive government program ever, according to numbers from Jim Bianco (as I saw it reported by Barry Ritholtz).
Bianco’s numbers are adjusted for inflation, which is good, but that isn’t a complete picture. There are an awful lot more Americans now than there used to be. If you look at the bailout in terms of per capita cost or as a percentage of GNP, you’ll see that there were a few other programs that were comparably expensive. So yeah, it’s bad. Yeah, it’s a big deal. But we have seen worse.
Program |
Inflation-adjusted cost (billions) |
Cost per capita (thousands) |
% of GNP |
Marshall Plan |
115.3 |
0.78 |
4.7% |
Savings and Loan crisis |
256 |
0.95 |
1.7% |
Moon shot |
237 |
1.2 |
4.3% |
Iraq war |
587 |
2.0 |
4.4% |
Korean war |
454 |
2.9 |
15.0% |
Vietnam war |
698 |
3.4 |
11.2% |
New Deal |
500 |
4.0 |
55.1% |
Current bailout |
4616 |
15.3 |
33.2% |
World War II |
3600 |
26.3 |
150% |
Louisiana Purchase |
217 |
40.9 |
NA |
Notes: It was surprisingly hard to find historical GNP figures. It only started being recorded in 1947, and the sources aren’t always clear if the figures are inflation-adjusted or not. Also, most of these things spanned several years; I picked a year near the middle for the calculations. Bottom line: take the % of GNP numbers with a grain of salt. They are close, but not exact.
I used the Flow of Funds Accounts of the United States for 1947-2007, and the a very poorly annotated list from Duke for the New Deal and WW2 numbers. Sorry, there are no GNP numbers from 1803.
Permalink
Posted in Gay rights, Politics at 1:08 pm by ducky
Salon has an interesting interview with Richard Rodriguez, who says — as I do — that the fight over “protecting traditional marriage” is really about protecting traditional gender roles. However, he spotted something that I missed: the role of male insecurity.
And the majority of American women are now living alone. We are raising children in America without fathers. I think of Michael Phelps at the Olympics with his mother in the stands. His father was completely absent. He was negligible; no one refers to him, no one noticed his absence.
The possibility that a whole new generation of American males is being raised by women without men is very challenging for the churches. I think they want to reassert some sort of male authority over the order of things. I think the pro-Proposition 8 movement was really galvanized by an insecurity that churches are feeling now with the rise of women.
I have been struck in the past at how when The Loyal Opposition talks about gay and lesbian people adopting, they usually emphasize, “a child needs a mother and a father”. It’s usually men I see saying this; Rodriguez’ interview makes me think that what they are really saying is, “Men are important! We are! We are! We are!”, trying to convince both us and themselves that it is true.
(By the way, children do just fine with same-gender parents.)
Permalink
Posted in Gay rights, Politics at 12:56 pm by ducky
Our Loyal Opposition in the marriage equality fight likes to yammer about how research shows that children do better when they are raised by both of their biological parents. This is utter hogwash.
The Loyal Opposition uses studies that show that children raised by both of their biological parents do better than those raised by a single parent. Studies comparing kids raised by a mixed-gender couple compared to those raised by a same-gender couple shows absolutely no difference on many many measures of success and well-being — delinquency, dropout rate, alcoholism, teen pregnancy, drug abuse, etc. By contrast, the difference between kids of two-parent families was absolutely huge compared to kids from single-parent families on all of the measures of success and well-being.
My source for the research on family structure effect on children’s well-being is an extensive longitudinal literature review that the Santa Clara County Social Services Agency did in 1996, a time when you would think society just might have made life even more difficult for gay and lesbian parents.
The only measure where there was any difference was a very very slight (but statistically significant) difference in sexual experimentation: children of gay/lesbian parents were no more likely to be gay/lesbian themselves, but they were very slightly more likely to experiment with homosexuality a few times.
While I am not familiar with any research on biological vs. non-biological two-parent families, it isn’t relevant. If there is a kid who needs adoption, their adoptive family won’t be their biological family, regardless of whether they get placed with a straight or homosexual couple.
I don’t know of any research that suggests that children of parents who used donated eggs or sperm are less happy than biological children. I suppose it could be true, but if it is, The Loyal Opposition should oppose infertility treatments of all kinds. Somehow I expect they wouldn’t take on that fight.
I know some people who think that gay and lesbian couples shouldn’t adopt because their children would face discrimination. By that logic, we shouldn’t allow black people to have children in the US; we shouldn’t allow Christians to have children in China.
Even if there were some difference between parenting by gay and lesbian couples and straight couples, that still isn’t an adequate reason to try and block their child-rearing. That’s a false comparison. The real comparison that you need to make is between children in foster care and those that get adopted. I suspect that adopted kids do far better than those that remain in foster care, and there is a surplus of kids to adopt.
While it is true that it is difficult to find healthy white babies to adopt, sadly, there are lots of non-white, non-healthy babies available. When my husband and I were going through foster parent training, Santa Clara County had seven times as many foster children as they had foster homes. SEVEN TIMES. (And you can be sure not every foster home took seven children!)
We should celebrate and encourage gay and lesbian adoption, not hinder it!
Permalink
11.20.08
Posted in Gay rights, Married life, Politics at 11:26 am by ducky
I found an anti-marriage-equality piece (via Andrew Sullivan) that was very interesting to me because of how it reflected its values.
I saw a striking example of what Jonathan Haight has found about differences in morality between liberals and conservatives. Haight found that conservatives are more likely to value “moral purity”, which basically says “if it feels icky to me, then it must be morally wrong”.
In his essay, Rod Dreher quotes University of Virginia sociologist James Davison Hunter:
“The momentum is toward experience and emotions and feelings. People are saying, ‘I feel, therefore I am.’ This is how more and more people are deciding what is real and right and true.”
Dreher complains that liberals don’t value that:
You can see this in the remarkable unwillingness of many gay-marriage defenders to grant their opponents any moral standing. To disagree with them is to reveal yourself to be a “bigot” (I heard a married, straight young Republican in Texas use that word to describe those who voted for Prop 8; he was far from the only one). Bigots are by definition people whose prejudices are irrational. Bigots are moral cretins who can’t be talked to, only coerced. One is under no obligation to compromise with a bigot, only to smash him.
I think he’s absolutely right. Liberals cannot understand the value that “if it feels icky, it must be wrong” (especially if “it” doesn’t feel icky to the liberals). Furthermore, there is no arguing with such a “moral purity” value. Joe Liberal cannot reason their way to making Joe Conservative feel less icky; Joe Liberal sees it as non-rational irrational because it is not rational by definition. It is emotional.
Dreher also laments the loss of the “meaning of marriage”:
Though no consensus on gay marriage now exists, the trend lines are not in traditionalists’ favor, in large part because our culture has lost its understanding of what marriage is for. That is, marriage no longer has a settled meaning beyond a nominalist one: it is a contract formalizing the positive emotions two people (for now) have for one another, and binding them in a legal and social framework.
I, a liberal, read that, and go, “yes, that is exactly what civil marriage is”. (I even have an old blog posting titled “Civil marriage is a contract“!)
Dreher doesn’t explain what the “meaning of marraige” is, but Andrew Sullivan (who perhaps is more familiar with Dreher’s corpus) says:
Rod longs, as many do, for a return to the days when civil marriage brought with it a whole bundle of collectively-shared, unchallenged, teleological, and largely Judeo-Christian, attributes. Civil marriage once reflected a great deal of cultural and religious assumptions: that women’s role was in the household, deferring to men; that marriage was about procreation, which could not be contracepted; that marriage was always and everywhere for life; that marriage was a central way of celebrating the primacy of male heterosexuality, in which women were deferent, non-heterosexuals rendered invisible and unmentionable, and thus the vexing questions of sexual identity and orientation banished to the catch-all category of sin and otherness, rather than universal human nature.
This is exactly what I was getting at in this post and in the first paragraph of this post. Marriage equality is not a threat to traditional marriage. It is a threat to traditional gender roles.
Permalink
Posted in Art, Random thoughts at 10:52 am by ducky
A while back, I wrote about LOLcats being a stand-in for ethnic groups, allowing us the humour of shared stereotypes but without having to saddle an ethnic group with those stereotypes.
Jay Dixit has a more expansive, romantic take on it: LOLcats are stand-ins for humans in all their glory and pathos. By being stand-ins, they are less emotionally dangerous:
By articulating profound feelings through cats and marine mammals speaking garbled English, we’re able to shroud genuine emotions in pseudo-irony — which means those animals can evoke deeper emotions without fear of mockery or cheapness.
I’ll put it more simply: humour is pain at a distance. Using cats (or dogs or walruses) lets us put even more distance between us and the pain. We can thus tolerate situations in LOLcats that would be too painful if it were about humans.
Hmm, I wonder if this is why animated cartoons so frequently starred animals (e.g. Mickey Mouse, Roadrunner, Foghorn Leghorn)…
Permalink
11.19.08
Posted in Email at 8:01 pm by ducky
I spoke a while back with Jason Gallic, the Product Marketing Manager for Email Center Pro. They have a product designed for improving email-based customer service, including automatic reply templates.
Fourteen years ago, I got to use a webmail system @ATS, developed for the National Center for Supercomputing Applications by the talented Ben Johnson. (Ben? Email me!) @ATS let you set up filters that would suggest a response if the condition you specified was met. When you read a message, after the message at the bottom, there would be a few checkboxes next to titles of suggested responses. I had the option of selecting any or none of the checkboxes, then pressing either a “Send as is” button or “Edit response” button. @ATS would include the responses that I checked, and send/let me edit it.
For example, I had one filter set up to suggest the “Undergraduate admissions” answer if the word “admissions” was in the body of the message. I had another filter which suggested the “Graduate admissions answer” if the word “admissions” was in the body of the message. By reading the message, I could sometimes tell if they were interested in graduate or undergraduate admissions, in which case I would click the appropriate box and send it on. Sometimes I couldn’t tell, so I would click both boxes and send it on. Sometimes I wanted to add a little extra information that I happened to know — if, for example, they asked about who would be a good advisor for research on hydrogen embrittlement in high-carbon steels — I would check the “graduate admissions” box and add the additional information before sending it on.
I ranted to Jason about how useful auto-suggest is; we’ll see if he manages to get it into his product.
Permalink
11.05.08
Posted in Politics at 5:03 pm by ducky
In his post today, Scott Rosenberg suggests that there are people who blame the blogosphere for how intensely nasty and partisan our political world is right now.
Excuse me????
Partisan nastiness has been going on far longer than people have been blogging. The Web was pretty well unknown during Clinton’s first term, and in its infancy during the second. I seem to recall a whole lot of partisan bickering back then.
I don’t think that divisiveness is due to the Web, I believe that it’s due to conservatives.
That’s a little hard for me to write because I want to be fair. But I really think it is true.
I recently read an article on research in morality that points up values differences between liberals and conservatives. One thing that researchers found was that liberals put a much higher value on fairness than on group loyalty, while conservatives value them about equally. This research suggests that a liberal is more likely to sacrifice group loyalty in the name of fairness than a conservative, e.g. to help a conservative do the work to send in an absentee ballot. This research suggests conservative is more likely to toe the party line, even if he/she doesn’t believe in it.
When Palin was insinuating that Barack Obama wasn’t a “real” American, she was exploiting her white audiences’ high value on group loyalty. By making it look like Obama had a different in-group, Palin made her audience worry that they might end up as the out-group.
(Being a member of an out-group might be particularly scary if you have yourself treated out-groups unfairly. I’m just sayin’.)
I was totally unconcerned about being in Obama’s out-group. You would think that I, a 45-year old, hot, white woman with an upper Midwest accent, who lives above the 48th parallel, might identify more strongly with Sarah Palin. However, I am a liberal, and I believe that Obama is a liberal. As such, I absolutely believe he will be fair. I absolutely do not believe that Palin will be fair. And I think that is part of her appeal to her base.
(P.S. I was kidding about being hot.)
Permalink
Posted in Canadian life, Family, Politics at 1:45 pm by ducky
Several people have asked me, “So are you and Jim moving back to California now?”
The answer is “No, not yet. Maybe never.”
I had six reasons to move to Canada:
- I was devastated that my fellow Americans could elect G.W. Bush for a second term. That said to me that my fellow Americans and I were not at all on the same page, and that maybe I didn’t belong in the US.
- I was upset at how my government shredded civil liberties for both citizens (e.g., illegal wiretapping) and non-citizens (e.g., torture and abuse).
- I was unnerved by an almost willful neglect/disinterest in some major, fundamental structural problems in the US and Californian economies. In particular, the US has been, as Lloyd Bentsen famously put it in a 1988 VP debate, been “writing hot checks” for a very long time: spending a lot but not paying enough in taxes to support those costs.
- UBC was more nurturing than Stanford, my other choice for grad school.
- We have lots of relatives close to Vancouver, just across the border in Bellingham.
- Canada’s health system is not tied to employment. It is highly likely that we will, at some point, earning money but not be employed. Living in Canada, that’s not a problem. (Like right now. I’m looking for work and Jim is consulting.) Living in the US, that might be a problem.
The fact that my compatriots turned out in such droves for Obama lessens the feeling that I am out of step with the rest of America. I was shocked and appalled by the divisive tactics used by the McCain/Palin campaign, but enormously heartened at the number of Republicans who have publicly voiced being likewise shocked and appalled. So Obama’s election knocks off #1 pretty well.
I have finished my graduate degree, so #4 is off the list.
Our families are still in Bellingham. We could move to Seattle and be slightly closer to our families, but California would be quite a bit farther away. So #5 favours Vancouver or Seattle, but still disfavours California.
I think Obama will probably make #2 better. Issuing an executive order banning torture at one minute past noon on Jan 20, 2009 would be a good start, but to see how he does on #2, I’ll have to see him govern.
Likewise, on #3, I won’t know if he will make things better until I see him govern. However, it’s not likely that he will be able to avoid “hot checks” in his term because of the horrible horrible financial problems. He also can’t do much about California’s problems due to Prop 13.
There are more factors to consider now.
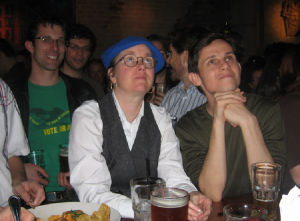
Ducky Watching Election Returns
- I like many things about Canada and Vancouver.
- I have friends here. (It was really nice to watch the election last night surrounded by a bunch of friends!)
- It is really cool to live in the heart of downtown. We are able to walk to everything (so much so that we only use our car about twice per month).
- I like, in theory, that there is skiing so close. We have season passes this year to a mountain that we can see from our apartment. It takes about 30 minutes to drive there.
- By and large, Canadian government services have far better customer service than in California. It takes me about twenty minutes to renew my Social Insurance Number (like a Social Security Number in the US). It took me about fifteen minutes to move my driver’s license to BC.
- It is not a perfect fit.
- In particular, I still have ambitions to change the world, while I think Vancouver puts more value on having fun. I’m trying to get the “fun” attitude, but it’s swimming upstream for me. (Hopefully the ski passes this winter will help!) Silicon Valley is all about changing the world, and so that is a huge magnet attracting me south.
- I don’t like maple syrup, I have never played hockey, and I thought Anne of Green Gables was a boring book. I did not spend many years steeped in the Canadian cultural stew, absorbing the Canadian value system, shared experiences, and etiquette. I will never be fully Canadian. (At the same time, the longer I stay in Canada, the less time I spend in the American cultural stew; the less American I become.)
- Somewhat to my surprise, I discovered that I still love my country.
- I am growing to love Canada.
- I haven’t found a job yet.
So. Will I return to the ever return to the US? To California? I’m not sure.
Permalink
« Previous entries Next Page » Next Page »